Loop Your Way to Java Mastery: Exploring the Art of Iteration
Imagine you’re sitting comfortably on your couch one evening, flipping through different TV channels in search of a movie to watch. You start by tuning into the first channel, hoping to find an exciting film. But, oh no! It’s a news channel discussing serious politics, not exactly what you were looking for. Just like you quickly press the remote button and move on to the next channel, in Java programming, you can use loops to swiftly navigate through a series of instructions. Loops allow you to repeat a certain block of code multiple times until a specific condition is met. They’re like the remote control of programming, helping you skip over unwanted parts and focus on what you really need.
This time, you come upon a cooking show on which a celebrity chef is showing delicious meals. It sparks your interest for a moment, but cooking isn’t your current drive, so you keep switching stations. You go through a series of options, including sports games, reality shows, wildlife documentaries, and even a late-night talk show.
You’ve set out to locate the ideal entertainment match! Finally, after several switches, you stumble upon a movie channel that captures your interest. It has the action, comedy, or drama you’ve been looking for. You settle in, grab your popcorn, and enjoy the movie.
This simple scenario beautifully demonstrates why loops are important in our daily lives. Just like your continuous channel surfing until you found the right show, loops in programming allow us to repeat a specific task until we achieve the desired result. Whether it’s searching for the right TV channel, organizing items, or analyzing data, loops provide a powerful tool for efficient and iterative operations.
In the world of Java programming, loops are like magical tools that allow you to automate repetitive tasks. They are designed to help you perform a set of instructions over and over again without having to write the same code repeatedly.
In this blog, we’ll look at the interesting world of Java loops. We’ll learn about each sort of loop, how they function, and how to utilize them successfully in your programs. So, let’s dive into the interesting world of Java loops.
Introduction To Loops In Java
Loops in Java are like a powerful magic trick that lets your program repeat a set of instructions as many times as needed. They save you from writing the same code over and over again and make your program run faster and smarter. Just like a loop in a roller coaster, your code goes through the loop again and again until it reaches the end. It’s a handy tool that helps you automate repetitive tasks and get things done more quickly and efficiently.

Let’s first understand the need for looping constructs in Java:-
Need for Looping constructs:
- Loop facilitates “WORM – Write Once, Run Multiple”- We don’t need to write the same code again and again, Just write once and run multiple times.
- They enable us to repeat the execution of a block of code based on particular conditions.
- Loops allow us to repeat through arrays, collections, or calculations a predetermined number of times.
- They let us regulate the flow of our program by executing code based on specified conditions.
- We may make our code more compact, adaptable, and powerful by using loops.
Elements of the Loops in Java:
When it comes to using loops in Java, there are a few essential elements you need to keep in mind. These elements form the building blocks of loops and enable you to control the repetition of code:
- Initialization Expression(s): Before starting a loop, you need to set up the initial conditions. This involves initializing a loop variable, which acts as a counter or a condition tracker. It determines how many times the loop will execute.
- Condition Expression(s): Loops rely on a condition to determine whether the loop should continue running or stop. This condition is evaluated before each iteration. If the condition is true, the loop continues; if it’s false, the loop terminates.
- Body of the Loop: Inside the loop, you define the code that should be repeated. This block of code is executed repeatedly until the condition becomes false. It’s where you perform the desired actions or calculations that need to be repeated.
- Update Expression(s): After each iteration, you need to update the loop variable or any other relevant variables. This step ensures that the loop progresses correctly and eventually terminates when the condition becomes false.

By understanding and properly utilizing these elements, you can create powerful and efficient loops in your Java programs. They allow you to control the flow of execution and handle repetitive tasks with ease.
Types of Loops In Java
- “For Loop”: Assume you have a particular activity to perform and you know how often you want to repeat it. Let’s say you want to count from 1 to 10. After each count, you raise the number by one, starting at one and continuing until you reach ten. Java’s for loop makes it simple to complete such repetitive jobs by allowing you to provide the initial value, the condition to continue, and the increment or decrement at the conclusion of each repetition.
- “While Loop”: It’s like telling your friend to keep doing something as long as a certain condition is true. For example, you might say, “Keep eating cookies until you run out of them.” As long as there are cookies left, your friend will keep munching on them.
- “Do-While Loop”: It’s similar to the “while loop,” but with a small twist. Imagine telling your friend, “Try playing the game at least once, and if you enjoy it, keep playing until you’re tired.” Your friend will try the game first, and if they enjoy it, they’ll keep playing until they’re tired.
For-Loop
When you have a task that needs to be repeated several times and you know how many times, like counting or printing numbers, writing the same code over and over again can be time-consuming and repetitive. Thankfully, in Java, we have a powerful tool called “For Loops” that helps us automate such repetitive tasks in a simple and efficient way.
A for loop is like a handy machine that takes care of performing a specific action multiple times for us. It has three important parts:
- Initialization: We set a starting point, like a counter, before the loop begins.
- Condition: We define a condition that determines whether the loop should continue or stop. It’s like a checkpoint that the loop checks each time.
- Increment/Decrement: We specify how the counter should change after each repetition, moving it closer to our desired outcome.
Once we set up these three parts, the for loop takes over and repeats the specified action until the condition becomes false. This saves us from writing the same code repeatedly, making our programs more concise and efficient.
For example, if we want to print numbers from 1 to 10, we can use a for loop in Java. We start at 1, check if the number is less than or equal to 10, print the number, and then move on to the next number. This process continues until we reach 10.
Syntax:
for (initialization condition; testing condition; increment/decrement)
{
statement(s)
}
Flow Diagram
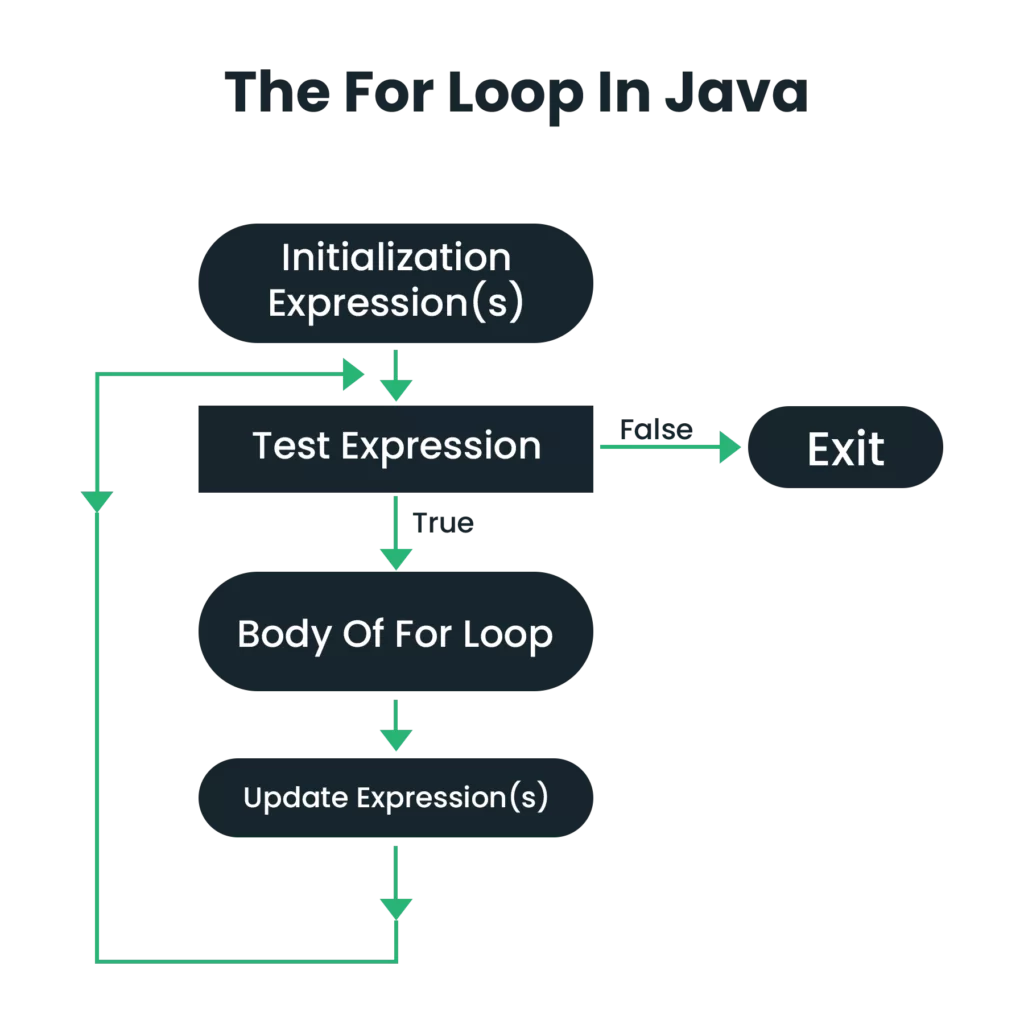
Working of For-Loops
Let’s understand the working of For-Loops with the help of the above example i.e. counting from 1 – 10. In Java, you can use a for loop to achieve this in a simple and efficient way.
Here’s how it works:
for (int number = 1; number <= 10; number++) {
System.out.println(number);
}
Now, let’s break it down step by step:
- First, you set up the for loop by specifying three things inside the parentheses:
- Initialization: You declare a variable called number and set it to 1. This is where your counting starts.
- Condition: You state that the loop should continue as long as number is less than or equal to 10. This ensures that you count up to 10 and then stop.
- Increment: You specify that after each iteration, the number variable should be incremented by 1. This is how you move from one number to the next.
- Inside the loop, you have the code that gets executed with each iteration. In this case, you use the System.out.println() statement to print the value of number to the console.
- The loop repeats the process until the condition is no longer met. So, it starts with number equal to 1, prints it, then increments number by 1. It continues this process until number reaches 10.
As a result, when you run this code, you’ll see the numbers 1 to 10 being printed on the screen, showcasing your amazing counting skills.
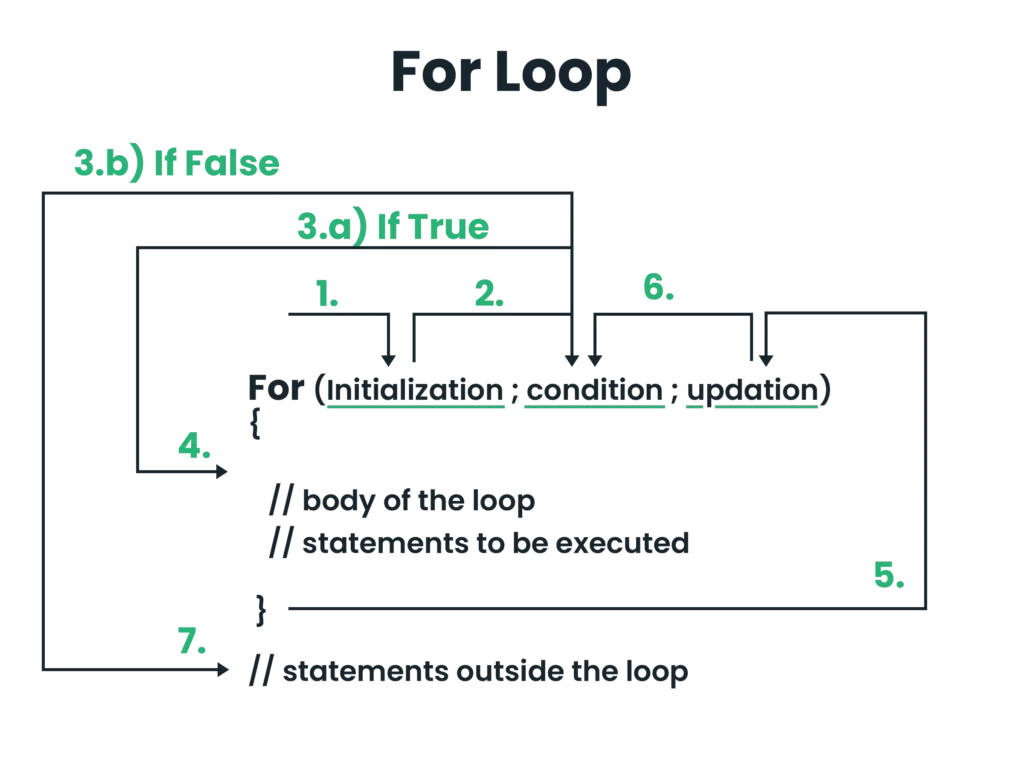
While Loops In Java
Imagine you have a task that you want to keep doing until a certain condition is met or you don’t know how many times you need to repeat the task to meet that condition. Maybe you want to keep eating ice cream as long as you’re not full or keep practicing a dance move until you get it just right. In Java, we have a handy tool called “While Loops” that allow us to do exactly that.
While loops are like friendly helper that keeps doing something over and over again until a specific condition becomes false. They are perfect for situations where we don’t know in advance how many times we need to repeat a task, but we have a condition to guide us.
For example, imagine you want to count from 1 to 5. You can use a while loop by starting with the number 1. As long as the number is less than or equal to 5, you increase it by 1 and say the number out loud. The loop keeps going until the number reaches 6, and then it stops.
While loops are great because they let us repeat actions without knowing in advance how many times we need to do them. They make our programs flexible and adaptable. Whether it’s processing data, checking user input, or controlling program flow, `while` loops come to the rescue.
Syntax
while (boolean condition)
{
loop statements...
}
Flow Diagram
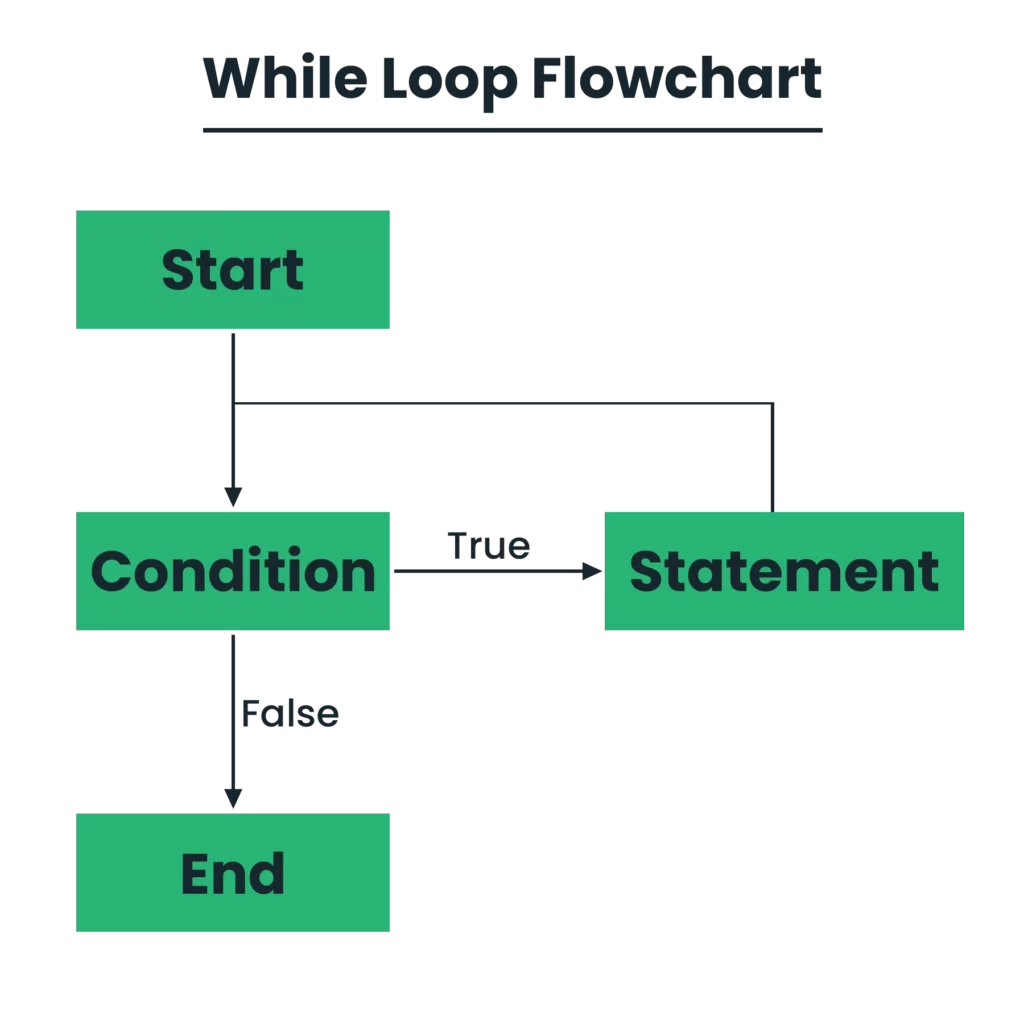
Working of While Loops
Imagine, you have a jar filled with candies but you don’t know how many candies are in there, so you started taking out one candy at a time and keeping the candy count.
Here’s how it works:
while (candiesInJar > 0) {
System.out.println("Eating a candy...");
candiesInJar--; // Reduce the count of candies by 1
}
System.out.println("No more candies left in the jar!");
Now let’s break it down step by step:
- There is a variable called “candiesInJar” and with some value already initialized, indicating the number of candies in the jar.
- The while loop condition checks if there are still candies in the jar (i.e., candiesInJar > 0).
- If the condition is true, it means there are candies remaining, and the loop body is executed.
- Inside the loop, we print the message “Eating a candy…” to indicate that we are consuming one candy.
- We then decrement the count of candies by 1 using the `candiesInJar–` statement.
- The loop continues to iterate as long as there are candies in the jar (i.e., the condition is true).
- Once there are no more candies left (i.e., candiesInJar becomes 0), the condition becomes false, and the loop exits.
- Finally, we print the message “No more candies left in the jar!” to indicate that we have consumed all the candies.
That’s how while loops work in Java! They provide a way to perform repetitive tasks that you don’t know how many times you need to repeat that task until a condition is no longer true.

Do-While Loops
Java’s Do-While loops are an effective tool that let us repeat an operation or set of actions up until a specific criterion is met. The distinguishing characteristic of a do-while loop is that it ensures that the code block will run at least once, whether the condition is true or false.
Think of it like this: Imagine you have a favorite ice cream shop that you want to visit. You enter the shop, and no matter what, you decide to have at least one scoop of your favorite ice cream. Once you finish the first scoop, you check if you want more. If you do, you keep getting more scoops until you are satisfied and decide not to have any more. Similar to this, a do-while loop begins by running the code block before checking the condition. The code block will be periodically executed until the condition is false if the condition is true. This guarantees that, like the initial scoop of ice cream, the code block is always run at least once.
Do-While loops in Java are especially useful when you need to perform an action that requires user input or validation. For example, you can prompt a user to enter some data, validate it, and then ask if they want to continue entering more data. If they do, the loop will repeat, allowing them to input more data until they decide to stop.
Syntax
do
{
statements..
}
while (condition);
Flow Diagram
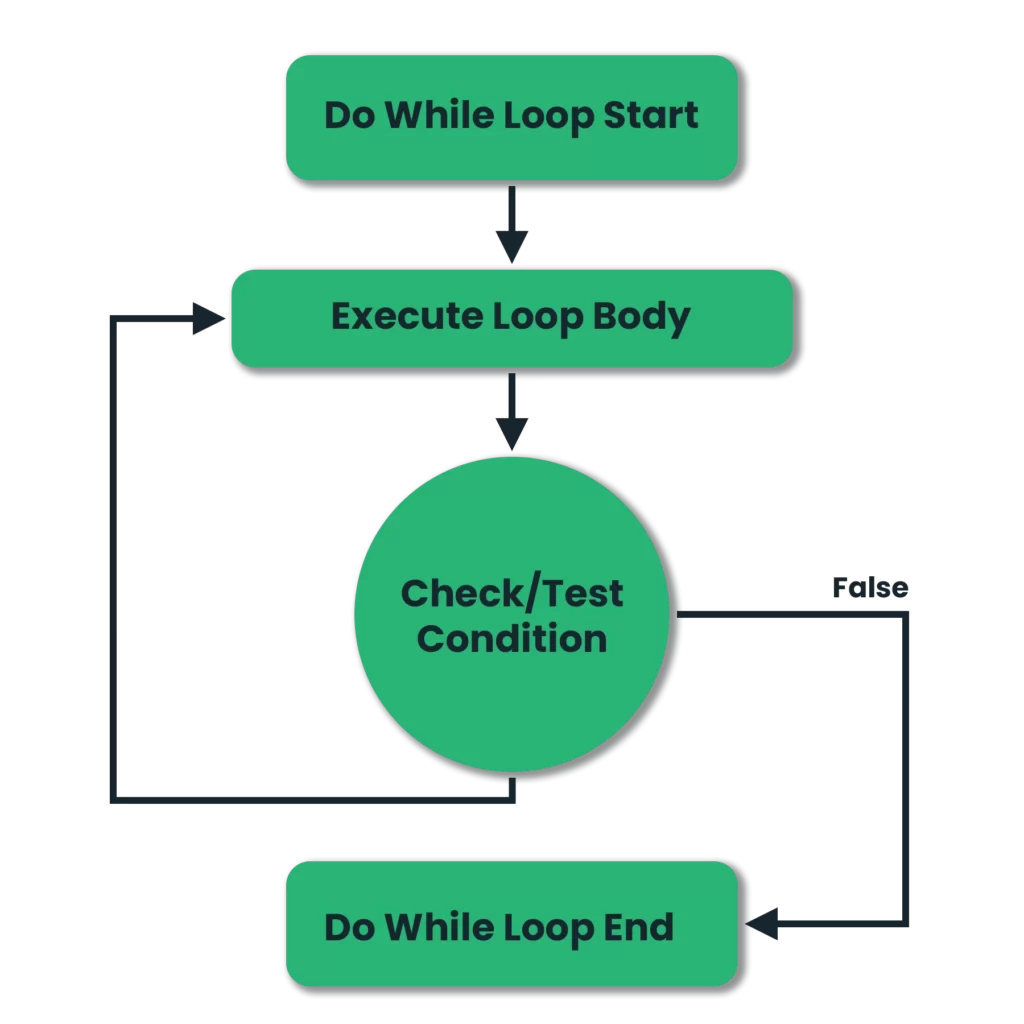
Working of Do-While Loops
Let’s understand the working of Do-While loops in Java with the help of an example. Imagine you are playing a guessing game. The game is having a secret number, and you have to guess what it is. You started by entering your first guess. The game would then check if the guess was correct. If it was, you would be congratulated, and the game would end. But if the guess was incorrect, the game would give a hint, telling you if your guess was too low or too high. The interesting part is that the game wouldn’t stop there. It gave you another chance to guess. This process would continue until you finally guessed the correct number.
This example can be demonstrated with the following code:
import java.util.Scanner;
public class GuessNumberGame {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int secretNumber = 42;
int guess;
do {
System.out.print("Guess the number (between 1 and 100): ");
guess = scanner.nextInt();
if (guess < secretNumber) {
System.out.println("Too low! Try again.");
} else if (guess > secretNumber) {
System.out.println("Too high! Try again.");
} else {
System.out.println("Congratulations! You guessed the correct number.");
}
} while (guess != secretNumber);
}
}
Here is how things are working in this :-
- Initialize variables: We set up the variables secretNumber as the target number to guess and guess to store the user’s guess.
- User input: The program prompts the user to enter a number and stores their guess in the guess variable.
- Comparison: The program compares the user’s guess with the secretNumber to determine if it is too low, too high, or correct.
- Output: Based on the comparison, the program provides feedback to the user, informing them if their guess is too low, too high, or correct.
- Loop execution: The Do-While Loop ensures that the user is prompted to enter another guess until they guess the correct number.
- Continuation or exit: If the user’s guess is incorrect, the loop continues, prompting the user to enter another guess. If the guess is correct, the loop exits and the game ends with a congratulatory message.
This process continues until the user guesses the correct number, and the loop then terminates.
In spite of whether the condition is initially true or not, the Do-While Loop always runs the code block at least once i.e. before checking whether the guessed number is a secret number or not, you first need to guess the number, and at least for once you need to guess a number. It offers a means of repeatedly carrying out operations based on user input up until a particular condition is met.
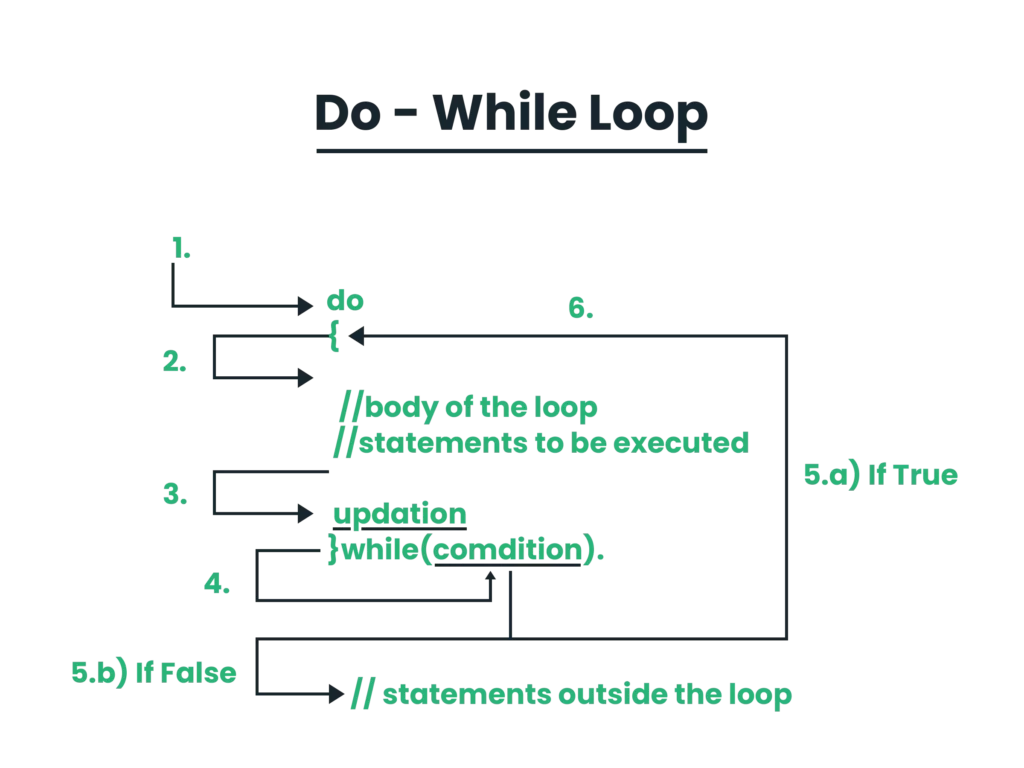
Till now, we have studied three different loops i.e. “For-loop”, “While-loop”, and “Do-While loop” and their features and when to use each of them. Now Let’s put some limelight on their key differences like how they are different from each other and when to use which loop.
Differences In For Loop And While Loop
- Structure: The for loop has three parts: initialization, condition, and iteration, like following a recipe with clear steps. The while loop has a simpler structure with just a condition, more like a repeated action based on a condition.
- Control: The for loop has built-in control, handling initialization and iteration within the loop. The while loop requires manual control, initializing variables before the loop and updating them inside the loop.
- Known Iterations: The for loop is used when you know the exact number of repetitions, like counting from one to ten. The while loop is more flexible, handling situations where the number of repetitions is uncertain, continuing until a certain condition is met.
- Flexibility: The while loop gives you more control over when to start and stop the loop based on a condition. The for loop, with its predefined structure, offers less flexibility but is useful when you have a fixed number of iterations in mind.
In summary, the for loop is like a recipe with clear steps for a known number of iterations. The while loop is a flexible repeated action based on a condition. Both loops have their strengths and can be used in different situations based on your program’s specific needs.

Differences Between While Loop And Do-While Loop
- Execution Flow: In a while loop, the condition is checked first, and if it is false, the loop is not executed at all. In contrast, a do-while loop executes the loop body at least once, regardless of the condition. It checks the condition after each execution.
- Condition Placement: In a while loop, the condition is placed at the beginning, controlling whether the loop should be executed or not. In a do-while loop, the condition is placed at the end, ensuring that the loop executes at least once before checking the condition.
- Loop Entry: The while loop may not execute at all if the initial condition is false. On the other hand, the do-while loop guarantees the execution of the loop body at least once, regardless of the initial condition.
- Loop Usage: The while loop is typically used when the number of iterations is uncertain and depends on a condition. It allows for flexibility in loop control. The do-while loop is useful when you want to ensure that a piece of code executes at least once before checking the condition.
In summary, `while` loops are suitable when the condition is evaluated before entering the loop, providing more control over whether the loop executes or not. `Do-while` loops guarantee the execution of the loop body at least once, making them useful when you want to ensure the code is executed before checking the condition. Both loops have their specific use cases based on the program’s requirements.
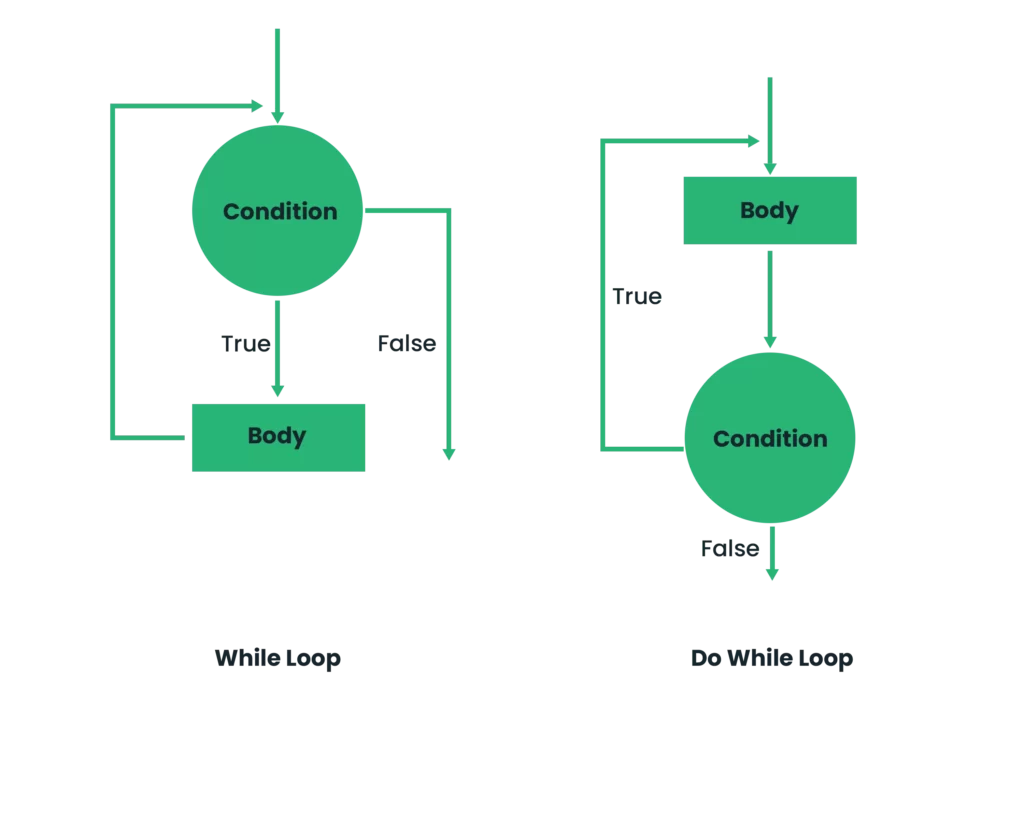
Differences Between For Loop And Do-While Loop
- Structure: The for loop has a compact structure with initialization, condition, and increment/decrement, while the do-while loop has a flexible structure with the loop body followed by the condition.
- Control: The for loop provides more control over loop execution, while the do-while loop guarantees the execution of the loop body at least once.
- Initialization: The for loop allows initializing the loop variable within the loop structure itself, while the do-while loop doesn’t have a dedicated initialization step.
- Usage: The for loop is commonly used when you know the number of iterations or want to iterate over a specific range of values, while the do-while loop is useful when you want to ensure that a piece of code executes at least once before checking the condition.
In simple terms, the for loop is like following a predefined plan, while the do-while loop is like trying something at least once before deciding whether to continue or not. Both loops have their unique purposes and can be chosen based on the specific requirements of your program.
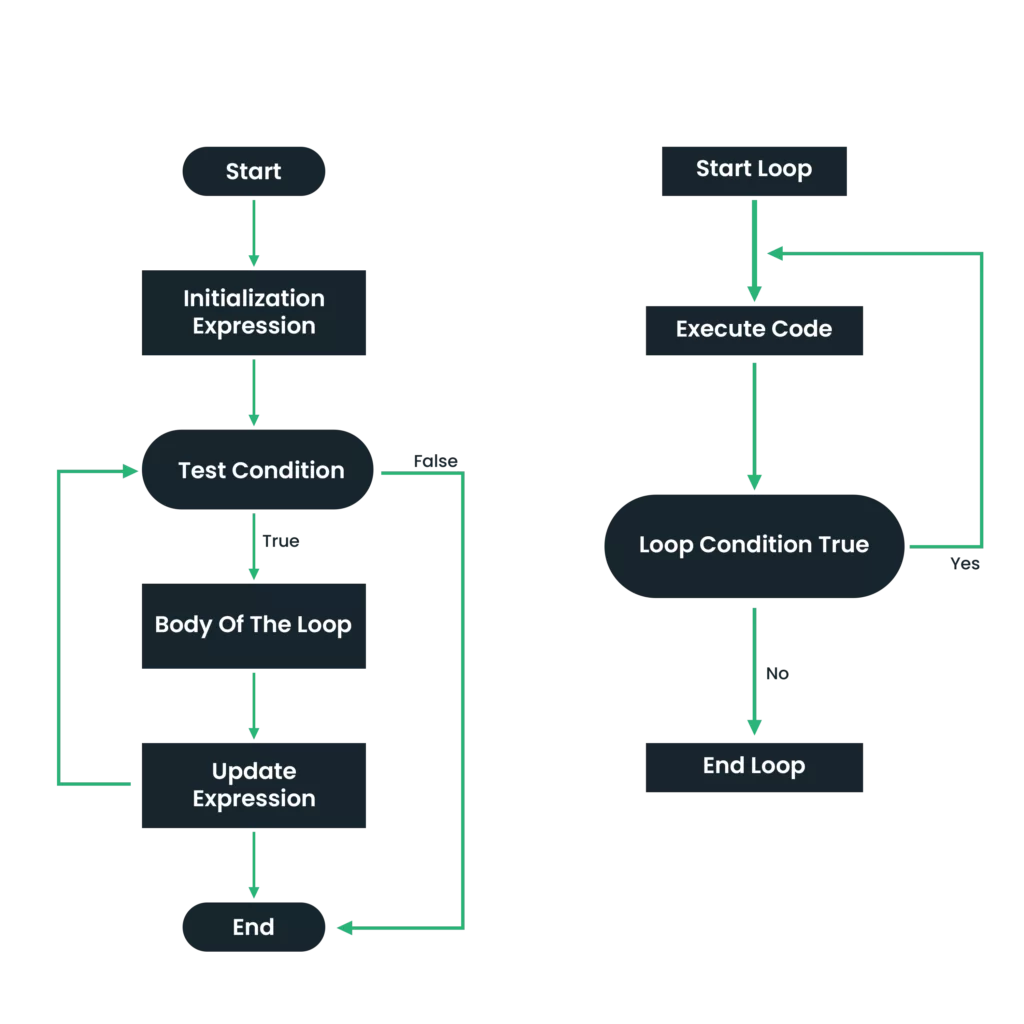
Continuing with the fascinating journey of Loops in Java, Let’s make some changes in the normal loops (After all change is the only constant). Think of the clock hanging on your room’s wall. Ever wondered how the hands of clock move and what pattern do they follow? The answer is hidden in the concept of nested loops in programming.
Nested Loops
As you observe the clock, you become curious about all the different time combinations it can display. How can you uncover this mystery?
Well, in Java programming, there’s something called nested loops that can help you solve this intriguing puzzle. Nested loops let you explore the various combinations of hours and minutes on the clock by moving the hands in a fascinating way. Think of it like a puzzle. The outer loop controls the hour’s hand, which can be set from 1 to 12. The inner loop controls the minute’s hand, which can be set from 0 to 59. By using nested loops, you can go through each hour and, within that, go through each minute. This way, you uncover every unique time combination the clock can display.
Just like the clock’s hands work together harmoniously, nested loops allow you to create a dynamic and intricate pattern of repetition. You can set up loops within loops, each controlling the motion of a specific hand. This arrangement unlocks a world of potential, enabling you to perform complex tasks with elegance and precision.
Nested loops in Java are loops within loops. They allow you to repeat actions in a structured way, like peeling layers of an onion. They’re useful for working with multiple variables and exploring complex patterns.
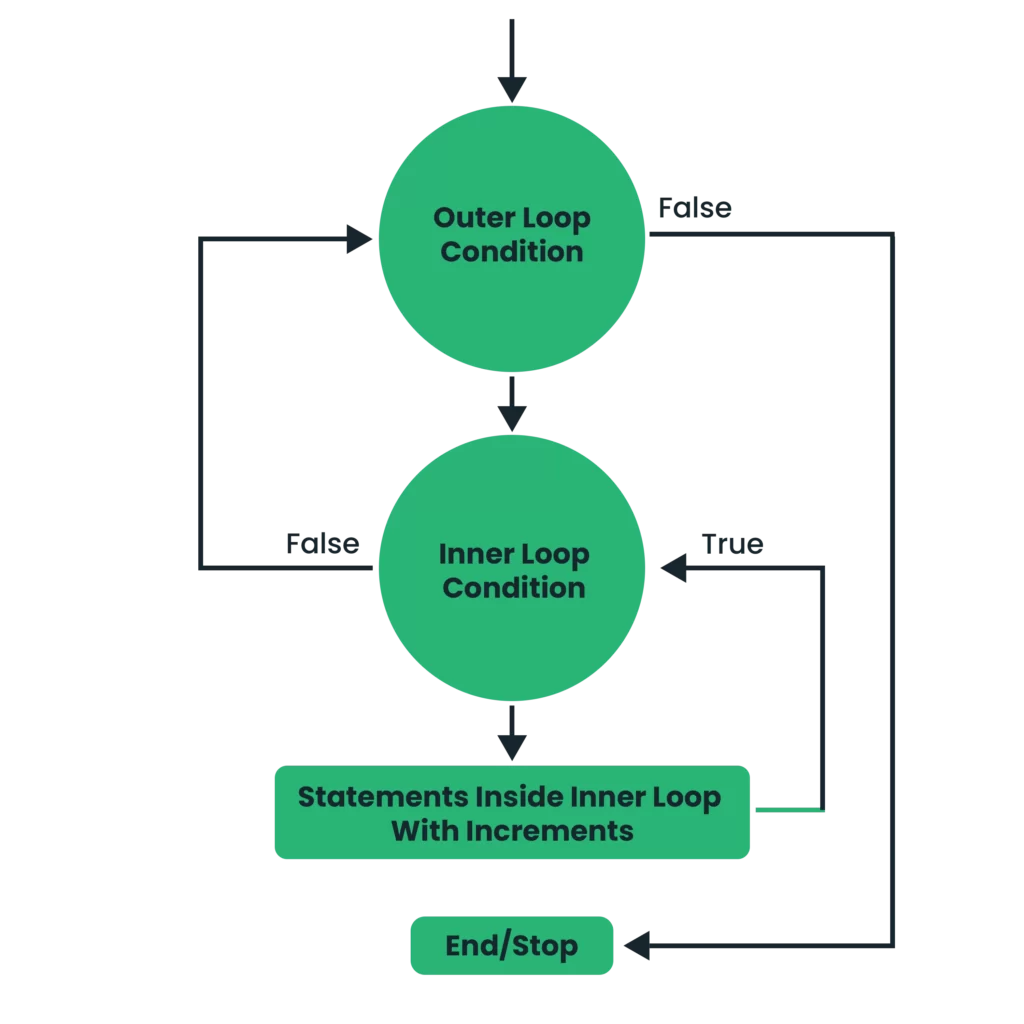
There are no restrictions on the type or sequence of loops; they can be nested with other loops of the same type or a different type. For instance, a while loop may be placed inside a for loop and vice versa. Likewise, a do-while loop may be placed inside another do-while loop. For example:
for (int i = 1; i <= 3; i++) {
int j = 1;
while (j <= 3) {
System.out.println("i: " + i + ", j: " + j);
j++;
}
}
Explanation:
- We have an outer loop, controlled by the variable `i`, which runs from 1 to 3 using a for loop.
- Inside the outer loop, we have an inner loop, controlled by the variable `j`, which runs from 1 to 3 using a while loop.
- The inner loop prints the values of `i` and `j` to the console.
- After each iteration of the inner loop, the variable `j` is incremented.
- Once the inner loop condition becomes false (`j` is no longer less than or equal to 3), the control goes back to the outer loop for the next iteration.
- The process continues until the outer loop completes all its iterations.
Output:
i: 1, j: 1
i: 1, j: 2
i: 1, j: 3
i: 2, j: 1
i: 2, j: 2
i: 2, j: 3
i: 3, j: 1
i: 3, j: 2
i: 3, j: 3
Note:- Not just that, you can do nesting of any number of loops, there is no restriction.
Variations In For-Loop
Multiple Initialization Expressions
Imagine you have a task where you need to keep track of two different things simultaneously. For example, you want to count the number of apples and oranges in a basket. With the multiple initializations variation, you can declare and initialize separate variables for each item right at the start of the loop. So, instead of just initializing a single variable like in a regular for loop, you can use a comma to separate the initializations of multiple variables. This way, you can conveniently set up and manage different counts or variables within the loop.
Here’s an example:
int apples, oranges;
for (apples = 0, oranges = 0; apples < 5 && oranges < 3; apples++, oranges++) {
// Code to count apples and oranges
}
In this example, we have two variables, apples and oranges, both initialized to 0. The loop will continue as long as the number of apples is less than 5 and the number of oranges is less than 3. Inside the loop, we can perform actions specific to each variable. By using multiple initializations in the for loop, you can conveniently handle multiple variables simultaneously and perform tasks that involve multiple entities or objects.
Multiple Update Expressions
Let’s imagine you are organizing a countdown event where you have two countdown clocks: one showing the countdown from 5 to 1, and the other showing the countdown from 10 to 0. To achieve this, you can use a for loop with multiple update expressions.
Code for the above:
for (int i = 1, j = 10; i <= 5; i++, j -= 2) {
System.out.println("Clock 1: " + i + ", Clock 2: " + j);
}
Explanation:
The code uses a for loop with two variables, i and j, to simulate a countdown on two clocks. The loop runs as long as i is less than or equal to 5. In each iteration of the loop, the value of i is incremented by 1, representing the countdown on the first clock. The value of j is decremented by 2, representing the countdown on the second clock. The current counts on both clocks are printed using the System.out.println() statement.
This allows you to create a countdown effect where one clock counts down from 5 to 1, and the other counts down from 10 to 0. By using multiple update expressions in the for loop, you can conveniently update and manipulate multiple variables, enabling you to achieve desired behaviors and calculations in your program.
Output:
Clock 1: 5
Clock 2: 10
Clock 1: 4
Clock 2: 8
Clock 1: 3
Clock 2: 6
Clock 1: 2
Clock 2: 4
Clock 1: 1
Clock 2: 2
Optional Expressions
In a for loop, the test expression, initialization expression, and update expression are all optional. This means that you can skip any or all of them based on your specific needs.
Here’s an example that demonstrates each of these expressions being skipped:
for (;;) {
// Code block to be executed
System.out.println("Hello, world!");
}
In this example, all three expressions are omitted. The loop will continue indefinitely, repeatedly executing the code block within the loop body. Since there is no test expression to evaluate and no update expression to modify the loop variable, the loop will run indefinitely until it is manually terminated.
Skipping the test expression, initialization expression, or update expression can be useful in certain scenarios. For example, if you want to create an infinite loop or if you have specific conditions outside of the loop that determine when to break out of the loop.
Enhanced For Loops
The for-each loop, commonly referred to as the extended for loop, is a practical Java syntax method for iterating through an array or collection. In comparison to conventional for loops, it offers a simplified syntax, making it simpler to work with data collections. Here’s how the enhanced for loop works:
for (datatype element : collection) {
// Code block to be executed
System.out.println(element);
}
Let’s break down the components of the enhanced for loop:
- datatype: This is the data type of the elements in the collection. It specifies the type of the variable element that will be used to access each element in the loop.
- element: This is a temporary variable that represents each individual element in the collection during each iteration of the loop. You can choose any valid variable name to represent the element.
- collection: This is the array or collection that you want to iterate over. It can be an array, a List, a Set, or any other data structure that implements the Iterable interface.
The enhanced for loop, or for-each loop, is a simplified way to iterate over elements in an array or collection in Java. It eliminates the need for manual indexing and provides a more concise syntax for iterating over data. It’s read-only, making it suitable for scenarios where you only need to access elements sequentially.
Empty Loop
An empty loop in Java is a loop structure where the loop body is intentionally left empty, but the initialization, test, and update expressions are included. It is often used to create delays or pause execution in programs.
Code example:
for (int i = 0; i < 10; i++) {
// Empty loop body
}
It’s important to note that an empty loop should be used with caution, as an infinite loop can lead to program freezing or unresponsive behavior. Therefore, it’s crucial to have a mechanism in place to break out of the loop when the desired condition is met.
Variations In While Loop
Multiple Condition Expression
In Java, you can use multiple condition expressions in a while loop to check multiple conditions simultaneously. By combining conditions using logical operators like “&&” (AND) or “||” (OR), you can create complex conditions that control the loop’s execution.
Example:
int age = 25;
boolean isStudent = true;
while (age < 30 && isStudent) {
// Loop body
age++;
}
In this example, the while loop continues executing as long as the age is less than 30 and the person is a student. Once any of the conditions becomes false, the loop will terminate.
This variation allows you to perform specific actions or iterations based on multiple conditions, providing flexibility in controlling the loop’s behavior.
Empty While Loop
In Java, an empty while loop is a loop structure that does not have any statements or code inside its body. It consists of the while keyword followed by parentheses, which contain the loop’s condition.
Example:
while (condition) {
// Empty loop body
}
An empty while loop evaluates the loop condition. Since there are no statements to change the condition, the loop will iterate endlessly if the condition is true. The loop will not be run at all if the condition is false.
This type of loop is commonly used when you want to create a loop that solely relies on the condition to control its execution. It can be useful in scenarios where you want to wait for a particular condition to become true or to create an infinite loop that can be terminated by other means, such as using a break statement.
Till now, we have studied various Loops and their variations. Now let’s see some pitfalls while writing loops in Java.
Pitfalls In Java
- Infinite loops:
while (true) {
// Code here
}
This code snippet creates an infinite loop because the loop condition is always true. Without a way to exit the loop, it will keep executing indefinitely, leading to a program that hangs or crashes.
- Off-by-one errors:
for (int i = 0; i < 5; i++) {
// Code here
}
In this example, the loop will execute 5 times, but the loop variable i will have values from 0 to 4. If you intended to include 5 in the loop, the condition should be i <= 5 instead of i < 5.
- Modifying loop variables:
for (int i = 0; i < 5; i++) {
// Code here
i += 2; // Modifying the loop variable
}
Modifying the loop variable i within the loop body can lead to unexpected behavior. In this case, the increment statement i += 2 can cause the loop to skip iterations and potentially result in an incorrect number of executions.
- Forgetting to update loop variables:
int i = 0;
while (i < 5) {
// Code here
}
In this example, the loop variable i is never updated inside the loop body. This will result in an infinite loop because the condition i < 5 will always be true, causing the loop to repeat indefinitely.
- Properly scoping variables:
int i;
for (i = 0; i < 5; i++) {
// Code here
}
// Code that uses i outside the loop
In this code snippet, the loop variable i is declared outside the loop, making it accessible outside the loop as well. This can lead to unintended side effects or confusion, as the variable may retain its value from the last iteration of the loop.
- Misplacing loop control statements:
for (int i = 0; i < 5; i++) {
// Code here
if (i == 2) {
break; // Misplaced break statement
}
}
In this example, the break statement is placed inside the loop, but it should be used to exit the loop when a certain condition is met. However, in this case, it will always break the loop when i equals 2, resulting in premature termination of the loop.
- Running out of memory with infinite loops:
ArrayList<Integer> list = new ArrayList<>();
while (true) {
list.add(1); // Adding elements infinitely
}
In this code snippet, an ArrayList is being populated inside an infinite loop. As elements are added continuously, memory usage keeps increasing until it eventually runs out of memory. This can cause a program to crash or become unresponsive.
- Unintended infinite loops:
int count = 0;
while (count < 10) {
// Some code here
count--;
}
In this example, the variable count is initialized to 0, and the loop condition is count < 10. However, within the loop body, count is decremented instead of incremented. This results in an unintended infinite loop where count never reaches the termination condition, leading to a program that gets stuck and doesn’t progress further. To avoid this pitfall, it’s important to carefully check the loop condition and ensure that the loop variable is updated in a way that allows the loop to eventually terminate.
By being aware of these pitfalls and avoiding them in your code, you can ensure that your loops behave as intended and produce the expected results.
Comparison Of For Loop, While Loop, And Do-While Loop
Factors | For Loop | While Loop | Do-While Loop |
Initialization | Initialization statement is present. | No initialization statement. | No initialization statement. |
Condition | Condition is checked before each iteration. | Condition is checked before each iteration. | Condition is checked after each iteration. |
Execution | Loop body may not execute if the condition is initially false. | Loop body may not execute if the condition is initially false. | Loop body always executes at least once, regardless of the condition. |
Iteration Count | Suitable for a known number of iterations. | Suitable for condition-based iterations. | Suitable for condition-based iterations. |
Loop Control | The loop control is integrated within the loop structure. | The loop control is explicitly written in the loop condition. | The loop control is explicitly written in the loop condition. |
Flexibility | Offers more flexibility for complex iterations. | Offers flexibility for condition-based iterations. | Offers flexibility for condition-based iterations. |
Preferred Usage | Suitable for iterating over a range of values. | Suitable for condition-based loops and indefinite loops. | Suitable for condition-based loops and indefinite loops. |
Syntax | `for (initialization; condition; update) { … }` | `while (condition) { … }` | `do { … } while (condition);` |
Conclusion
- Loops in Java are used to repeat a block of code multiple times, making it easier to perform repetitive tasks.
- The for loop is suitable when you know how many times you want to repeat the code and need to control the loop with an initialization, condition, and update.
- The while loop is used when you want to repeat the code as long as a specific condition is true.
- The do-while loop is similar to the while loop but guarantees that the code executes at least once before checking the condition.
- It’s important to ensure that the loop conditions are correctly defined and updated to avoid infinite loops.
- You can combine and nest loops to create more complex repetitive patterns.
- Choosing the right loop construct depends on the specific requirements of your program and the control flow you want to achieve.
Practice and experimentation with loops are key to becoming proficient in using them effectively. Using loops efficiently can simplify your code, improve performance, and enhance the functionality of your Java programs.
Happy Looping!